Attached below is a Bash shell script used to push photographs into Drupal 10. We are running a time-lapse project with Drupal serving as the back-end repository. Using Drupal's out-of-the-box REST services makes for an easy setup. Multiple cameras can be quickly integrated, making a very scalable solution if you want to run a lot of cameras at once. Drupal would actually make a pretty nice security cam dashboard with a little tweaking to this code.
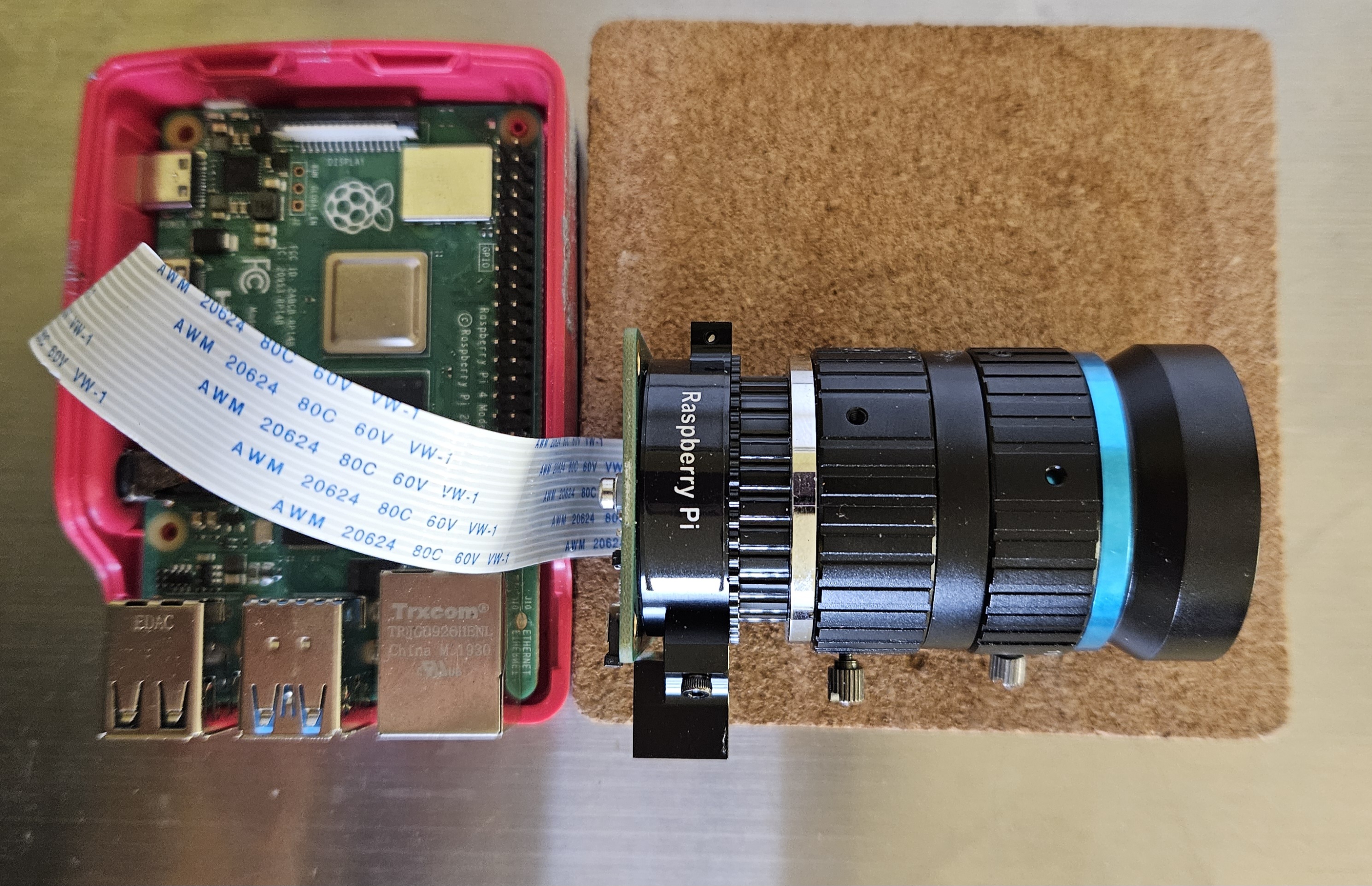
#!/bin/bash
source config.sh
# Capture and process the image
libcamera-still -o /camera/original.jpg
convert /camera/original.jpg -resize 1280x720 -quality 85 /camera/processed.jpg
# Upload the original image file
upload_file() {
local file_path=$1
local file_name=$(basename "$file_path")
local content_disposition="attachment; filename=\"$file_name\""
local response=$(curl -X POST "$FILE_UPLOAD_ENDPOINT" \
-u "$USERNAME:$PASSWORD" \
-H "Content-Type: application/octet-stream" \
-H "Content-Disposition: $content_disposition" \
--data-binary "@$file_path")
echo $response | grep -o '"fid":\[[^]]*\]' | grep -o '[0-9]\+'
}
# Upload the original file and capture its ID
file_id=$(upload_file "/camera/processed.jpg")
create_media() {
local file_id=$1
local media_data=$(cat <<EOF
{
"bundle":[{"target_id":"image"}],
"name":[{"value":"Raspberry Pi Image"}],
"field_media_image":[{"target_id":"$file_id"}]
}
EOF
)
echo "Media Data: $media_data"
# Execute the curl command and capture the response
local response=$(curl -X POST "$MEDIA_ENDPOINT" \
-u "$USERNAME:$PASSWORD" \
-H "Content-Type: application/json" \
-d "$media_data")
echo "Raw Media Creation Response: $response"
# Extract and return the media ID from the response
local media_id=$(echo $response | grep -o '"mid":\[[^]]*\]' | grep -o '[0-9]\+')
echo "Extracted Media ID: $media_id"
}
create_media "$file_id"
# Create Media entity and capture its Media ID
media_response=$(create_media "$file_id")
media_id=$(echo $media_response | grep -o '"mid":\[[^]]*\]' | grep -o '[0-9]\+')
echo "Captured Media ID: $media_id"
# Prepare JSON data for creating the node with the Media entity
node_data=$(cat <<EOF
{
"type": [{"target_id": "test_file_upload"}],
"title": [{"value": "Raspberry Pi High Def Camera Project"}],
"field_media_image": [{"target_id": "$media_id"}]
}
EOF
)
echo "JSON Payload for Node Creation: $node_data"
# Create the node
node_response=$(curl -v -X POST "$NODE_ENDPOINT" \
-u "$USERNAME:$PASSWORD" \
-H "Content-Type: application/json" \
-d "$node_data")
echo "Response from Node Creation: $node_response"
# EXAMPLE for the source config.sh file
USERNAME="chaneg_me"
PASSWORD="change_me"
FILE_UPLOAD_ENDPOINT="https://example.com/file/upload/node/test_file_upload/field_test_upload"
MEDIA_ENDPOINT="https://example.com/entity/media"
NODE_ENDPOINT="https://example.com/node"
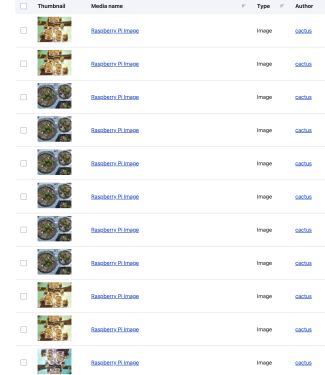